02-MyBatis-环境搭建
MyBatis 源码
MyBatis官方文档
源码下载地址(推荐使用码云中转下载)
mybatis-parent项目下载地址
- 选择自己所需要的MyBatis版本下载
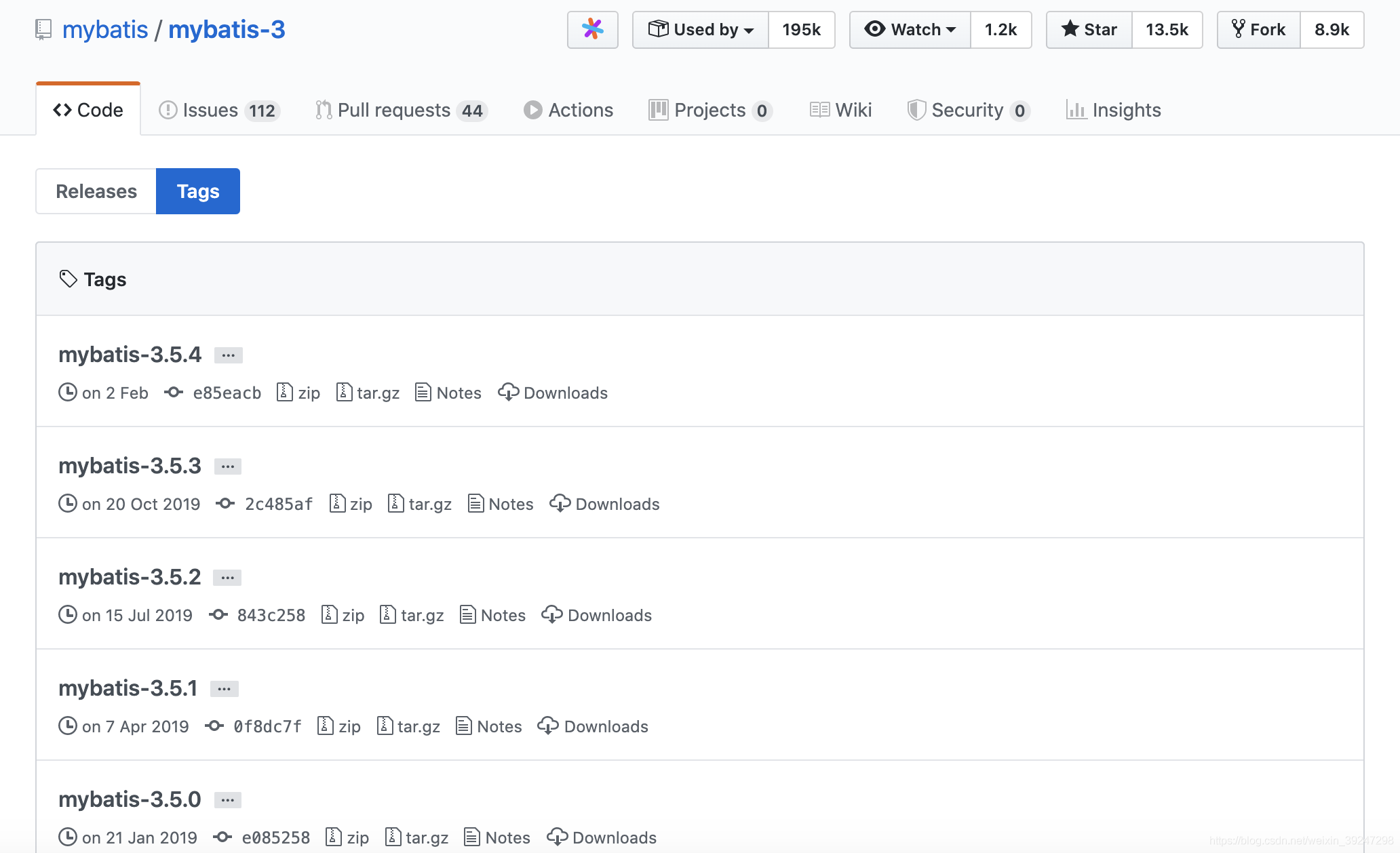
- 查看pom文件中所对应的mybatis-parent项目版本
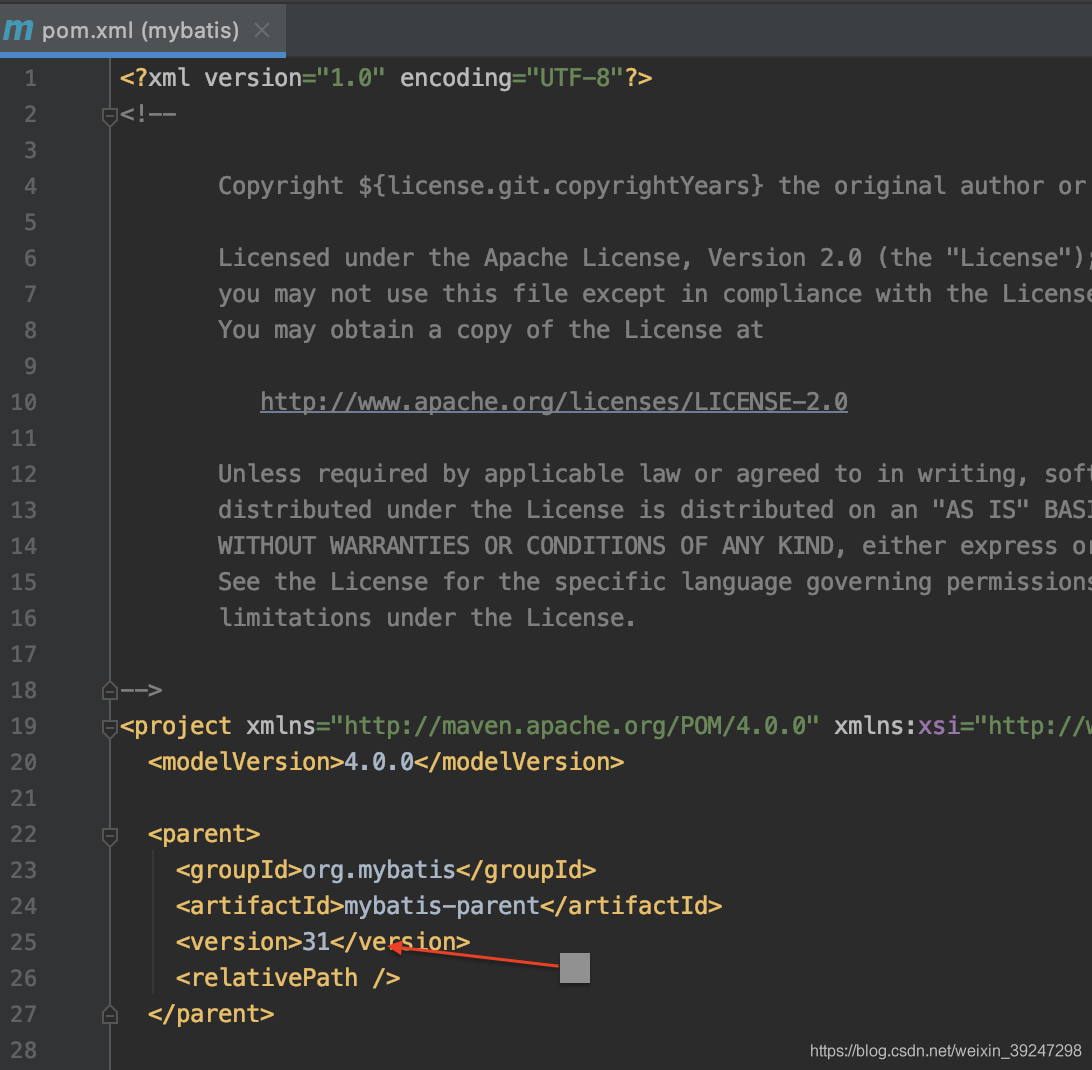
- 根据tag下载所需要的项目包。
- 导入idea,选择jdk进行编译。
- 新建一个maven模块
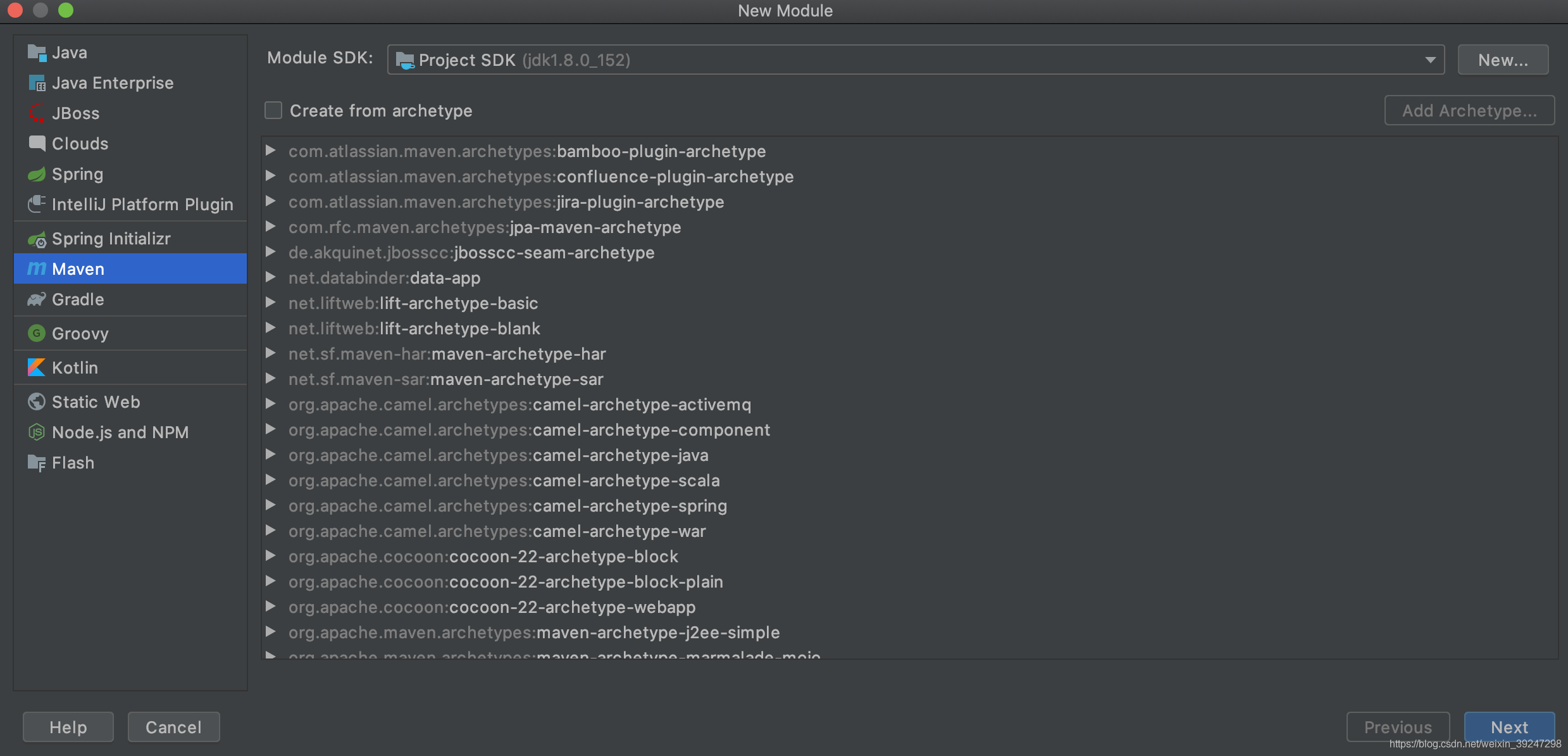
pom文件引入依赖
1 |
|
- 项目结构
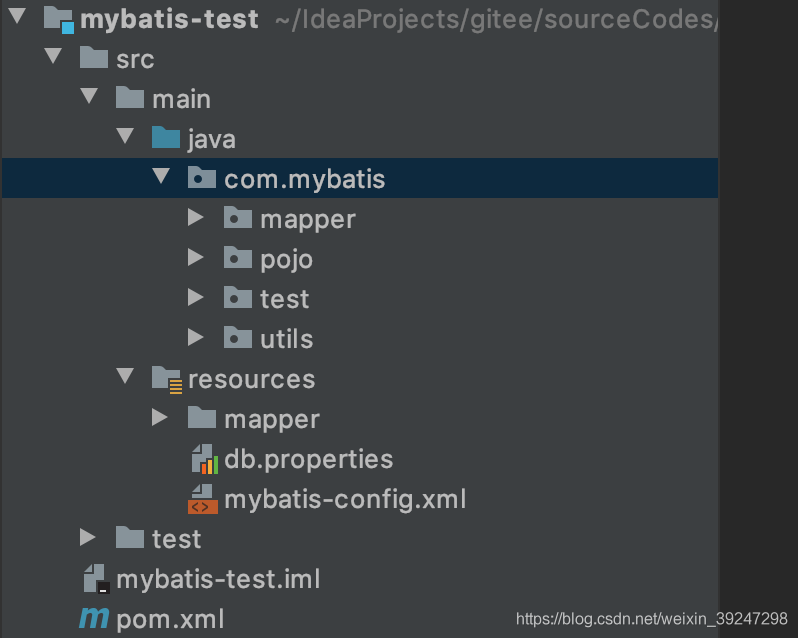
1 |
|
1 |
|
1 |
|
1 |
|
1 |
|
1 |
|
1 |
|
- 运行TestMybatis01中的select(),结果如图所示
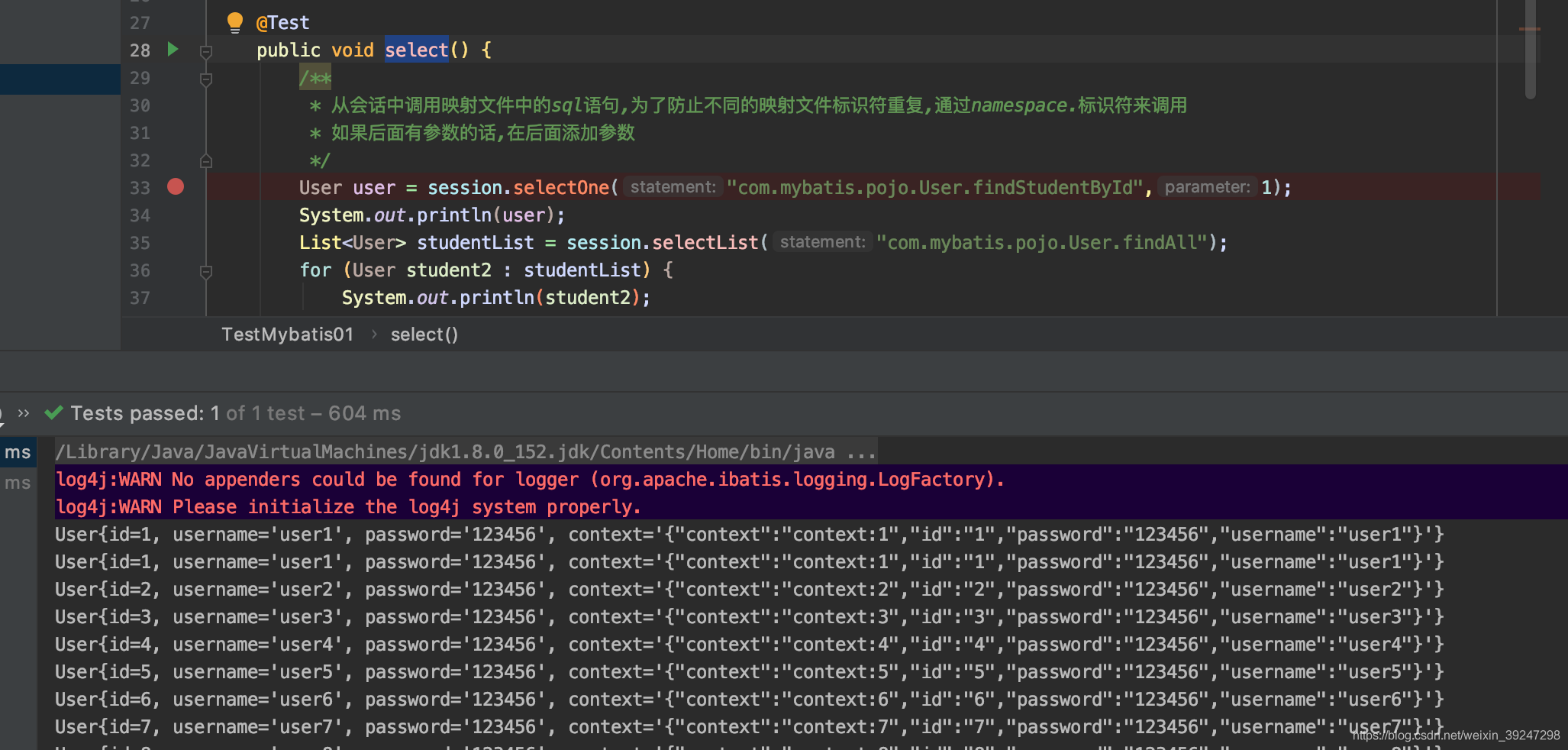
至此,mybatis源码阅读项目建立成功。
本博客所有文章除特别声明外,均采用 CC BY-SA 4.0 协议 ,转载请注明出处!